function blur_image ($image, $level
{
// based on http://www.tuxradar.com/practicalphp/11/2/25
$imagex = imagesx$image);
$imagey = imagesy$image);
$tc = imageistruecolor$image);
$new_image = imagecreatetruecolor$imagex, $imagey);
imagealphablending$new_image, false);
imagesavealpha$new_image, true);
$level = $level-1;
for ($x = 0; $x < $imagex; ++$x) {
for ($y = 0; $y < $imagey; ++$y) {
$newr = 0;
$newg = 0;
$newb = 0;
$newt = 0;
$colours = array();
$thiscol = imagecolorat$image, $x, $y);
for ($k = $x - $level; $k <= $x + $level; ++$k) {
for ($l = $y - $level; $l <= $y + $level; ++$l) {
if ($k < 0) { $colours[] = $thiscol; continue; }
if ($k >= $imagex) { $colours[] = $thiscol; continue; }
if ($l < 0) { $colours[] = $thiscol; continue; }
if ($l >= $imagey) { $colours[] = $thiscol; continue; }
$colours[] = imagecolorat$image, $k, $l);
}
}
foreach$colours as $colour) {
if ($tc) {
$newr += ($colour >> 16) & 0xFF;
$newg += ($colour >> 8) & 0xFF;
$newb += $colour & 0xFF;
$newt += ($colour >> 24) & 0xFF;
} else {
$c = imagecolorsforindex$image, $colour);
$newr += $c'red'];
$newg += $c'green'];
$newb += $c'blue'];
$newt += $c'alpha'];
}
}
$numelements = count$colours);
$newr /= $numelements
$newg /= $numelements
$newb /= $numelements
$newt /= $numelements
$newcol = imagecolorallocatealpha$new_image, $newr, $newg, $newb, $newt);
imagesetpixel$new_image, $x, $y, $newcol);
}
}
return $new_image
}
eXorithm – Execute Algorithm: View / Run Algorithm blur_image
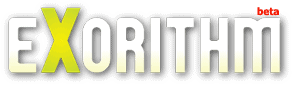