function draw_sphere ($image_size, $degree_x, $degree_y, $degree_z, $vdist, $dist, $vertex_color, $face_color, $wireframe, $dashes, $sphere_detail
{
$lats = 2*$sphere_detail+1; // latitudes, including equator and poles (should be odd)
$longs = $sphere_detail*4;
$degree_per_lat = 90/floor$lats/2);
$degree_per_long = 360/$longs
$radius = 200; // radius is arbitary
// build all the polygons that make up a sphere
for ($i=0;$iceil$lats/2);$i++) {
$y = $radius * cosdeg2rad$degree_per_lat$i));
$inner_radius = $radius * sindeg2rad$degree_per_lat$i));
for ($j=0;$j$longs$j++) {
$x = $inner_radius * sindeg2rad$degree_per_long$j));
$z = $inner_radius * cosdeg2rad$degree_per_long$j));
if ($iceil$lats/2)-1) {
$poly = $i$longs$j+1;
$sides$poly][6] = $x
$sides$poly][7] = $y
$sides$poly][8] = $z
$sides$poly*-1][6] = $x
$sides$poly*-1][7] = $y*-1;
$sides$poly*-1][8] = $z
if ($i>0) {
$poly = $i$longs+(($j$longs-1) % $longs)+1;
$sides$poly][9] = $x
$sides$poly][10] = $y
$sides$poly][11] = $z
$sides$poly*-1][9] = $x
$sides$poly*-1][10] = $y*-1;
$sides$poly*-1][11] = $z
}
}
if ($i>0) {
$poly = ($i-1)*$longs$j+1;
$sides$poly][3] = $x
$sides$poly][4] = $y
$sides$poly][5] = $z
$sides$poly*-1][3] = $x
$sides$poly*-1][4] = $y*-1;
$sides$poly*-1][5] = $z
$poly = ($i-1)*$longs+(($j$longs-1) % $longs)+1;
$sides$poly][0] = $x
$sides$poly][1] = $y
$sides$poly][2] = $z
$sides$poly*-1][0] = $x
$sides$poly*-1][1] = $y*-1;
$sides$poly*-1][2] = $z;
}
}
}
$sides = array_values$sides);
// project each of the polygons
for ($i=0; $icount$sides); $i++) {
$points[] = project_polygon$sides$i], $degree_x, $degree_y, $degree_z, 0, 0, 0, $vdist$radius, $dist$radius, true);
}
// scale the image somewhat
$scale = $image_size/($radius*2.2);
return render_polygons$points, $vertex_color, $face_color, $wireframe, $dashes, $image_size, $scale);
}
eXorithm – Execute Algorithm: View / Run Algorithm draw_sphere
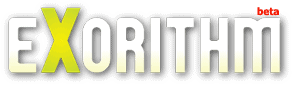