function equilateral_shape ($radius, $sides, $color
{
// blank image
$image = image_create_alpha$radius*2+4, $radius*2+4);
// create the color
$r = hexdecsubstr$color, 0, 2));
$g = hexdecsubstr$color, 2, 2));
$b = hexdecsubstr$color, 4, 2));
$color = imagecolorallocate$image, $r, $g, $b);
// The fudge factor is only used to rotate the shape so a flat
// line is at the bottom.
if (($sides%2)==0) {
if (($sides/2%2)==0) {
$fudge = pi()*1/$sides
} else {
$fudge = 0;
}
} else {
if ((($sides-1)/2%2)==0) {
$fudge = pi()*1.5/$sides
} else {
$fudge = pi()*0.5/$sides
}
}
$x0 = 0;
$y0 = 0;
// for the number of sides...
for ($i=0; $i$sides; $i++) {
// compute a point on the perimeter $i/$sides from the beginning
$x1 = round$radiuscos(2*pi()*$i$sides$fudge)+$radius+2);
$y1 = round$radiussin(2*pi()*$i$sides$fudge)+$radius+2);
if ($i==0) {
// If this is the first point then we can't draw a line yet
// because we don't have a second set of points to connect to.
// However, we need we need these points to connect the last
// set of points to.
$x0 = $x1
$y0 = $y1
} else {
// draw a line
imageline$image, $x1, $y1, $x2, $y2, $color);
}
// remember these points
$x2 = $x1
$y2 = $y1
}
// draw the final line
imageline$image, $x2, $y2, $x0, $y0, $color);
return $image
}
eXorithm – Execute Algorithm: View / Run Algorithm equilateral_shape
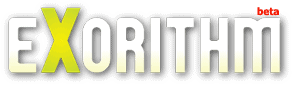