function pixelate ($image, $blocksize
{
// Based on http://www.tuxradar.com/practicalphp/11/2/24
$imagex = imagesx$image);
$imagey = imagesy$image);
$image2 = imagecreatetruecolor$imagex, $imagey);
imagesavealpha$image2, true);
imagealphablending$image2, false);
if ($blocksize<1) {
$blocksize = 1;
}
for ($x = 0; $x < $imagex; $x += $blocksize) {
for ($y = 0; $y < $imagey; $y += $blocksize) {
// get the pixel colour at the top-left of the square
$colour = imagecolorat$image, $x, $y);
// set the new red, green, blue and alpha values to 0
$newr = 0;
$newg = 0;
$newb = 0;
$newt = 0;
// create an empty array for the colours
$colours = array();
// cycle through each pixel in the block
for ($k = $x; $k < $x + $blocksize; ++$k) {
for ($l = $y; $l < $y + $blocksize; ++$l) {
// if we are outside the valid bounds of the image, use a safe colour
if ($k < 0) { $colours[] = $colour; continue; }
if ($k >= $imagex) { $colours[] = $colour; continue; }
if ($l < 0) { $colours[] = $colour; continue; }
if ($l >= $imagey) { $colours[] = $colour; continue; }
// if not outside the image bounds, get the colour at this pixel
$colours[] = imagecolorat$image, $k, $l);
}
}
// cycle through all the colours we can use for sampling
foreach$colours as $colour) {
$colour = imagecolorsforindex$image, $colour);
// add their red, green, and blue values to our master numbers
$newr += $colour'red'];
$newg += $colour'green'];
$newb += $colour'blue'];
$newt += $colour'alpha'];
}
// now divide the master numbers by the number of valid samples to get an average
$numelements = count$colours);
$newr /= $numelements
$newg /= $numelements
$newb /= $numelements
$newt /= $numelements
// and use the new numbers as our colour
$newcol = imagecolorallocatealpha$image2, $newr, $newg, $newb, $newt);
imagefilledrectangle$image2, $x, $y, $x + $blocksize - 1, $y + $blocksize - 1, $newcol);
}
}
return $image2
}
eXorithm – Execute Algorithm: View / Run Algorithm pixelate
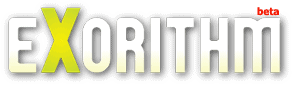