function project_polygon ($points, $degree_x, $degree_y, $degree_z, $center_x, $center_y, $center_z, $dist1, $dist2, $include_z
{
  // check points
  if ((count$points)%3)!=0) {
    throw new Exception'The points must be a list like x1, y1, z1, x2, y2, z2, etc. The number of points therefore must be divisible by three.');
  }
 Â
  $degree_x = deg2rad$degree_x);
  $degree_y = deg2rad$degree_y);
  $degree_z = deg2rad$degree_z);
 Â
  $cosx = cos$degree_x);
  $sinx = sin$degree_x);
  $cosy = cos$degree_y);
  $siny = sin$degree_y);
  $cosz = cos$degree_z);
  $sinz = sin$degree_z);
 Â
  $array = array();
 Â
  for ($i=0;$icount$points);$i$i+3) {
    $x0 = $points$i]-$center_x
    $y0 = $points$i+1]-$center_y
    $z0 = $points$i+2]-$center_z
   Â
    $x1 = $cosy*($sinz$y0 + $cosz$x0) - $siny$z0
    $y1 = $sinx*($cosy$z0 + $siny*($sinz$y0 + $cosz$x0)) + $cosx*($cosz$y0 - $sinz$x0);
    $z1 = $cosx*($cosy$z0 + $siny*($sinz$y0 + $cosz$x0)) - $sinx*($cosz$y0 - $sinz$x0);
 Â
    $x2 = $x1$dist1/($z1$dist1$dist2);
    $y2 = $y1$dist1/($z1$dist1$dist2);
    $z2 = $z1$dist1/($z1$dist1$dist2);
 Â
    $array[] = $x2
    $array[] = $y2
    if ($include_z) $array[] = $z2
  }
 Â
  return $array
}Â
eXorithm – Execute Algorithm: View / Run Algorithm project_polygon
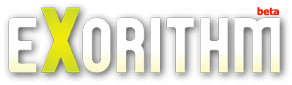