function raise_polygon ($points, $height, $direction, $color
{
// check points
if ((count$points)==0) || ((count$points)%2)!=0)) {
throw new Exception'The points must be an array with an even number of integers.');
}
// determine the extent of the polygon
$maxx = $points[0];
$maxy = $points[1];
$minx = $points[0];
$miny = $points[1];
for ($i=2; $icount$points); $i$i+2) {
$x = $points$i];
$y = $points$i+1];
if ($x$maxx) $maxx = $x
if ($y$maxy) $maxy = $y
if ($x$minx) $minx = $x
if ($y$miny) $miny = $y
}
// determine the extent of the projection
$proj_length = roundsqrt$height$height/2));
switch ($direction) {
case 'nw'
$projx = $proj_length*-1;
$projy = $proj_length*-1;
break
case 'ne'
$projx = $proj_length
$projy = $proj_length*-1;
break
case 'sw'
$projx = $proj_length*-1;
$projy = $proj_length
break
default
$projx = $proj_length
$projy = $proj_length
}
// determine where the polygon should be positioned
for ($i=0; $icount$points); $i$i+2) {
$points$i] = $points$i]-$minx+1;
$points$i+1] = $points$i+1]-$miny+1;
if ($projx<0) $points$i] = $points$i] - $projx
if ($projy<0) $points$i+1] = $points$i+1] - $projy
}
// blank image
$image = image_create_alpha$maxx$minx$proj_length+2, $maxy$miny$proj_length+2);
// create the colors
$r = hexdecsubstr$color, 0, 2));
$g = hexdecsubstr$color, 2, 2));
$b = hexdecsubstr$color, 4, 2));
$color = imagecolorallocate$image, $r, $g, $b);
// draw the first polygon
imagepolygon$image, $points, count$points)/2, $color);
// draw the lines + modify the points
for ($i=0; $icount$points); $i$i+2) {
imageline$image, $points$i], $points$i+1], $points$i]+$projx, $points$i+1]+$projy, $color);
$points$i] = $points$i]+$projx
$points$i+1] = $points$i+1]+$projy
}
// draw the second polygon
imagepolygon$image, $points, count$points)/2, $color);
return $image
}
eXorithm – Execute Algorithm: View / Run Algorithm raise_polygon
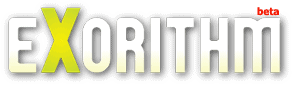