function rounded_rectangle ($height, $width, $corner_radius, $rectangle_color, $background_color, $background_transparent
{
if (($corner_radius>($height/2)) || ($corner_radius>($width/2))) {
throw new Exception'Corner radius may not exceed half the length of any of the sides.');
}
$image = image_create_alpha$width, $height);
if (!$background_transparent) {
$background_color = allocate_color$image, $background_color);
imagefilledrectangle$image, 0, 0, $width, $height, $background_color);
}
$rectangle_color = allocate_color$image, $rectangle_color);
// draw 2 rectangles that overlap (making an image like the cross on the Swiss flag)
imagefilledrectangle$image, $corner_radius, 0, $width$corner_radius-1, $height, $rectangle_color);
imagefilledrectangle$image, 0, $corner_radius, $width, $height$corner_radius-1, $rectangle_color);
// draw 4 circles for the corners
imagefilledellipse$image, $corner_radius, $corner_radius, $corner_radius*2, $corner_radius*2, $rectangle_color);
imagefilledellipse$image, $width$corner_radius-1, $corner_radius, $corner_radius*2, $corner_radius*2, $rectangle_color);
imagefilledellipse$image, $corner_radius, $height$corner_radius-1, $corner_radius*2, $corner_radius*2, $rectangle_color);
imagefilledellipse$image, $width$corner_radius-1, $height$corner_radius-1, $corner_radius*2, $corner_radius*2, $rectangle_color);
return $image
}
eXorithm – Execute Algorithm: View / Run Algorithm rounded_rectangle
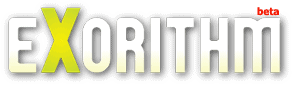