function simplify_equation ($equation
{
if (is_array$equation)) {
// first simply the parameters of the equation
$equation[1] = simplify_equation$equation[1]);
if (isset$equation[2])) {
$equation[2] = simplify_equation$equation[2]);
}
// simplify based on the operator
switch ($equation[0]) {
case '+'
if ((is_numeric$equation[1])) && (is_numeric$equation[2]))) {
return $equation[1]+$equation[2];
}
if ($equation[1]===0) {
return $equation[2];
}
if ($equation[2]===0) {
return $equation[1];
}
return $equation
case '-'
if ($equation[1]===$equation[2]) {
return 0;
}
if ((is_numeric$equation[1])) && (is_numeric$equation[2]))) {
return $equation[1]-$equation[2];
}
if ($equation[1]===0) {
return array'neg', $equation[2]);
}
if ($equation[2]===0) {
return $equation[1];
}
return $equation
case '*'
if ((is_numeric$equation[1])) && (is_numeric$equation[2]))) {
return $equation[1]*$equation[2];
}
if ($equation[1]===0) {
return 0;
}
if ($equation[2]===0) {
return 0;
}
if ($equation[1]===1) {
return $equation[2];
}
if ($equation[2]===1) {
return $equation[1];
}
return $equation
case '/'
if ($equation[2]===0) {
throw new Exception'division by zero');
}
if ($equation[1]===$equation[2]) {
return 1;
}
if ((is_numeric$equation[1])) && (is_numeric$equation[2]))) {
if (($equation[1] % $equation[2])==0) {
return $equation[1]/$equation[2];
} else {
// get the greastest common divisor
$gcd = gcd_euclid$equation[1], $equation[2]);
$equation[1] = $equation[1]/$gcd
$equation[2] = $equation[2]/$gcd
}
}
if ($equation[1]===0) {
return 0;
}
if ($equation[2]===1) {
return $equation[1];
}
return $equation
case '^'
if ((is_numeric$equation[1])) && (is_numeric$equation[2]))) {
return pow$equation[1], $equation[2]);
}
if ($equation[1]===0) {
return 0;
}
if ($equation[1]===1) {
return 1;
}
if ($equation[2]===0) {
return 1;
}
if ($equation[2]===1) {
return $equation[1];
}
return $equation
case 'neg'
if (is_array$equation[1])) {
if ($equation[1][0]=='neg') {
return $equation[1][1];
}
}
if (is_numeric$equation[1])) {
return $equation[1]*-1;
}
return $equation
case 'sqrt'
if (is_numeric$equation[1])) {
return sqrt$equation[1]);
}
return $equation
case 'log'
if ($equation[1]===1) {
return 0;
}
if ((is_numeric$equation[1])) && (is_numeric$equation[2]))) {
return log$equation[1], $equation[2]);
}
return $equation
case 'ln'
if (is_numeric$equation[1])) {
return log$equation[1]);
}
return $equation
case 'exp'
if (is_numeric$equation[1])) {
return exp$equation[1]);
}
return $equation
case 'root'
if ((is_numeric$equation[1])) && (is_numeric$equation[2]))) {
return pow$equation[1], 1/$equation[2]);
}
if ($equation[1]===0) {
return 0;
}
if ($equation[1]===1) {
return 1;
}
if ($equation[2]===0) {
throw new Exception'division by zero');
}
if ($equation[2]===1) {
return $equation[1];
}
return $equation
// trig
case 'sin'
if (is_numeric$equation[1])) {
return sin$equation[1]);
}
return $equation
case 'cos'
if (is_numeric$equation[1])) {
return cos$equation[1]);
}
return $equation
case 'tan'
if (is_numeric$equation[1])) {
return tan$equation[1]);
}
return $equation
case 'sec'
if (is_numeric$equation[1])) {
return 1/cos$equation[1]);
}
return $equation
case 'csc'
if (is_numeric$equation[1])) {
return 1/sin$equation[1]);
}
return $equation
case 'cot'
if (is_numeric$equation[1])) {
return 1/tan$equation[1]);
}
return $equation
// hyperbolic trig
case 'sinh'
if (is_numeric$equation[1])) {
return sinh$equation[1]);
}
return $equation
case 'cosh'
if (is_numeric$equation[1])) {
return cosh$equation[1]);
}
return $equation
case 'tanh'
if (is_numeric$equation[1])) {
return tanh$equation[1]);
}
return $equation
case 'sech'
if (is_numeric$equation[1])) {
return 1/cosh$equation[1]);
}
return $equation
case 'csch'
if (is_numeric$equation[1])) {
return 1/sinh$equation[1]);
}
return $equation
case 'coth'
if (is_numeric$equation[1])) {
return 1/tanh$equation[1]);
}
return $equation
// arc trig
case 'arcsin'
if (is_numeric$equation[1])) {
return asin$equation[1]);
}
return $equation
case 'arccos'
if (is_numeric$equation[1])) {
return acos$equation[1]);
}
return $equation
case 'arctan'
if (is_numeric$equation[1])) {
return atan$equation[1]);
}
return $equation
// inverse hyperbolic trig
case 'arsinh'
if (is_numeric$equation[1])) {
return asinh$equation[1]);
}
return $equation
case 'arcosh'
if (is_numeric$equation[1])) {
return acosh$equation[1]);
}
return $equation
case 'artanh'
if (is_numeric$equation[1])) {
return atanh$equation[1]);
}
return $equation
default
throw new Exception'usupported operator '$operator);
}
}
return $equation
}
eXorithm – Execute Algorithm: View / Run Algorithm simplify_equation
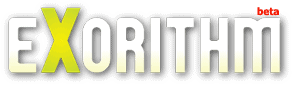