function skew_image ($image, $skew_value
{
// note: this function was original posted by etienne on php.net.
$width = imagesx$image);
$height = imagesy$image);
$height2 = $height + ($width * $skew_value);
$imgdest = image_create_alpha$width, $height2);
// Process the image
for$x = 0, $level = 0; $x < $width - 1; $x++)
{
$floor = floor$level);
// To go faster, some lines are being copied at once
if ($level == $floor
imagecopy$imgdest, $image, $x, $level, $x, 0, 1, $height - 1);
else
{
$temp = $level - $floor
// The first pixel of the line
// We get the color then apply a fade on it depending on the level
$color1 = imagecolorsforindex$image, imagecolorat$img, $x, 0));
$alpha = $color1'alpha'] + ($temp * 127);
if ($alpha < 127)
{
$color = imagecolorallocatealpha$imgdest, $color1'red'], $color1'green'], $color1'blue'], $alpha);
imagesetpixel$imgdest, $x, $floor, $color);
}
// The rest of the line
for$y = 1; $y < $height - 1; $y++)
{
// Merge this pixel and the upper one
$color2 = imagecolorsforindex$image, imagecolorat$image, $x, $y));
$alpha = ($color1'alpha'] * $temp) + ($color2'alpha'] * (1 - $temp));
if ($alpha < 127)
{
$red = ($color1'red'] * $temp) + ($color2'red'] * (1 - $temp));
$green = ($color1'green'] * $temp) + ($color2'green'] * (1 - $temp));
$blue = ($color1'blue'] * $temp) + ($color2'blue'] * (1 - $temp));
$color = imagecolorallocatealpha$imgdest, $red, $green, $blue, $alpha);
imagesetpixel$imgdest, $x, $floor + $y, $color);
}
$color1 = $color2
}
// The last pixel of the line
$color1 = imagecolorsforindex$image, imagecolorat$image, $x, $height - 1));
$alpha = $color1'alpha'] + ((1 - $temp) * 127);
if ($alpha < 127)
{
$color = imagecolorallocatealpha$imgdest, $color1'red'], $color1'green'], $color1'blue'], $alpha);
imagesetpixel$imgdest, $x, $floor + $height - 1, $color);
}
}
// The line is finished, the next line will be lower
$level += $skew_value
}
// Finished processing, return the skewed image
return $imgdest
}
eXorithm – Execute Algorithm: View / Run Algorithm skew_image
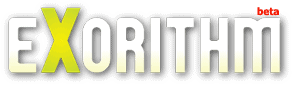